- Object Relational Mapping module:
- Hierarchical structure
- Constraints consistency and validation
- Object metadata depends on its status
- Optimised processing by complex query (multiple actions at once)
- Default field values
- Permissions optimisation
- Persistent object: DB postgresql
- Data conversion
- Multi-level caching system
- Two different inheritance mechanisms
- Rich set of field types:
- classical (varchar, integer, boolean, …)
- relational (one2many, many2one, many2many)
- functional
Models
Model fields are defined as attributes on the model itself:
from odoo import models, fields
class AModel(models.Model):
_name = 'a.model.name'
field1 = fields.Char()
Warning
this means you cannot define a field and a method with the same name, the last one will silently overwrite the former ones.
By default, the field’s label (user-visible name) is a capitalized version of
the field name, this can be overridden with the string
parameter.
field2 = fields.Integer(string="Field Label")
For the list of field types and parameters, see the fields reference.
Default values are defined as parameters on fields, either as a value:
name = fields.Char(default="a value")
or as a function called to compute the default value, which should return that value:
def _default_name(self):
return self.get_value()
name = fields.Char(default=lambda self: self._default_name())
API
class odoo.models.BaseModel[source]
Base class for Odoo models.
Odoo models are created by inheriting one of the following:
Model
for regular database-persisted modelsTransientModel
for temporary data, stored in the database but automatically vacuumed every so oftenAbstractModel
for abstract super classes meant to be shared by multiple inheriting models
The system automatically instantiates every model once per database. Those instances represent the available models on each database, and depend on which modules are installed on that database. The actual class of each instance is built from the Python classes that create and inherit from the corresponding model.
Every model instance is a “recordset”, i.e., an ordered collection of
records of the model. Recordsets are returned by methods like
browse()
, search()
, or field accesses. Records have no
explicit representation: a record is represented as a recordset of one
record.
To create a class that should not be instantiated,
the _register
attribute may be set to False.
_auto = False
Whether a database table should be created (default: True
).
If set to False
, override init()
to create the database table.
Tip
To create a model without any table, inherit
from AbstractModel
.
_table = None
SQL table name used by model if _auto
_sequence = None
SQL sequence to use for ID field
_sql_constraints = []
SQL constraints [(name, sql_def, message)]
_register = True
not visible in ORM registry
_name = None
the model name (in dot-notation, module namespace)
_description = None
the model’s informal name
_inherit = None
Python-inherited models:
_inherits = {}
dictionary {‘parent_model’: ‘m2o_field’} mapping the _name of the parent business objects to the names of the corresponding foreign key fields to use:
_inherits = {
'a.model': 'a_field_id',
'b.model': 'b_field_id'
}
implements composition-based inheritance: the new model exposes all the fields of the inherited models but stores none of them: the values themselves remain stored on the linked record.
Warning
if multiple fields with the same name are defined in the
_inherits
-ed models, the inherited field will
correspond to the last one (in the inherits list order).
_rec_name = None
field to use for labeling records, default: name
_order = 'id'
default order field for searching results
_check_company_auto = False
On write and create, call _check_company
to ensure companies
consistency on the relational fields having check_company=True
as attribute.
_parent_name = 'parent_id'
the many2one field used as parent field
_parent_store = False
set to True to compute parent_path field.
Alongside a parent_path
field, sets up an indexed storage
of the tree structure of records, to enable faster hierarchical queries
on the records of the current model using the child_of
and
parent_of
domain operators.
_abstract = True
whether model is abstract
See also
_transient = False
whether model is transient
See also
_date_name = 'date'
field to use for default calendar view
_fold_name = 'fold'
field to determine folded groups in kanban views
AbstractModel
odoo.models.AbstractModel[source]
alias of odoo.models.BaseModel
Model
class odoo.models.Model[source]
Main super-class for regular database-persisted Odoo models.
Odoo models are created by inheriting from this class:
class user(Model):
...
The system will later instantiate the class once per database (on which the class’ module is installed).
TransientModel
class odoo.models.TransientModel[source]
Model super-class for transient records, meant to be temporarily persistent, and regularly vacuum-cleaned.
A TransientModel has a simplified access rights management, all users can create new records, and may only access the records they created. The superuser has unrestricted access to all TransientModel records.
Fields
class odoo.fields.Field[source]
The field descriptor contains the field definition, and manages accesses and assignments of the corresponding field on records. The following attributes may be provided when instanciating a field:
- string (
str
) – the label of the field seen by users; if not set, the ORM takes the field name in the class (capitalized). - help (
str
) – the tooltip of the field seen by users - readonly (
bool
) –whether the field is readonly (default:
False
)This only has an impact on the UI. Any field assignation in code will work (if the field is a stored field or an inversable one).
- required (
bool
) – whether the value of the field is required (default:False
) - index (
bool
) – whether the field is indexed in database. Note: no effect on non-stored and virtual fields. (default:False
) - default (
value
or
callable
) – the default value for the field; this is either a static value, or a function taking a recordset and returning a value; usedefault=None
to discard default values for the field - states (
dict
) –a dictionary mapping state values to lists of UI attribute-value pairs; possible attributes are:
readonly
,required
,invisible
.Warning
Any state-based condition requires the
state
field value to be available on the client-side UI. This is typically done by including it in the relevant views, possibly made invisible if not relevant for the end-user. - groups (
str
) – comma-separated list of group xml ids (string); this restricts the field access to the users of the given groups only - company_dependent (
bool
) –whether the field value is dependent of the current company;
The value isn’t stored on the model table. It is registered as [UNKNOWN NODE title_reference]. When the value of the company_dependent field is needed, an [UNKNOWN NODE title_reference] is searched, linked to the current company (and current record if one property exists).
If the value is changed on the record, it either modifies the existing property for the current record (if one exists), or creates a new one for the current company and res_id.
If the value is changed on the company side, it will impact all records on which the value hasn’t been changed.
- copy (
bool
) – whether the field value should be copied when the record is duplicated (default:True
for normal fields,False
forone2many
and computed fields, including property fields and related fields) - store (
bool
) – whether the field is stored in database (default:True
,False
for computed fields) - group_operator (
str
) –aggregate function used by
read_group()
when grouping on this field.Supported aggregate functions are:
array_agg
: values, including nulls, concatenated into an arraycount
: number of rowscount_distinct
: number of distinct rowsbool_and
: true if all values are true, otherwise falsebool_or
: true if at least one value is true, otherwise falsemax
: maximum value of all valuesmin
: minimum value of all valuesavg
: the average (arithmetic mean) of all valuessum
: sum of all values
- group_expand (
str
) –function used to expand read_group results when grouping on the current field.
@api.model def _read_group_selection_field(self, values, domain, order): return ['choice1', 'choice2', ...] # available selection choices. @api.model def _read_group_many2one_field(self, records, domain, order): return records + self.search([custom_domain])
Computed Fields
- compute (
str
) –name of a method that computes the field
See also
- compute_sudo (
bool
) – whether the field should be recomputed as superuser to bypass access rights (by defaultTrue
for stored fields,False
for non stored fields) - inverse (
str
) – name of a method that inverses the field (optional) - search (
str
) – name of a method that implement search on the field (optional) - related (
str
) –sequence of field names
See also
Basic Fields
class odoo.fields.Boolean[source]
Encapsulates a bool
.
class odoo.fields.Char[source]
Basic string field, can be length-limited, usually displayed as a single-line string in clients.
- size (
int
) – the maximum size of values stored for that field - trim (
bool
) – states whether the value is trimmed or not (by default,True
). Note that the trim operation is applied only by the web client. - translate (
bool
or
callable
) – enable the translation of the field’s values; usetranslate=True
to translate field values as a whole;translate
may also be a callable such thattranslate(callback, value)
translatesvalue
by usingcallback(term)
to retrieve the translation of terms.
class odoo.fields.Float[source]
Encapsulates a float
.
The precision digits are given by the (optional) digits
attribute.
class odoo.fields.Integer[source]
Encapsulates an int
.
Advanced Fields
class odoo.fields.Binary[source]
Encapsulates a binary content (e.g. a file).
bool
) – whether the field should be stored as [UNKNOWN NODE title_reference]
or in a column of the model’s table (default: True
).class odoo.fields.Html[source]
Encapsulates an html code content.
- sanitize (
bool
) – whether value must be sanitized (default:True
) - sanitize_tags (
bool
) – whether to sanitize tags (only a white list of attributes is accepted, default:True
) - sanitize_attributes (
bool
) – whether to sanitize attributes (only a white list of attributes is accepted, default:True
) - sanitize_style (
bool
) – whether to sanitize style attributes (default:True
) - strip_style (
bool
) – whether to strip style attributes (removed and therefore not sanitized, default:False
) - strip_classes (
bool
) – whether to strip classes attributes (default:False
)
class odoo.fields.Image[source]
Encapsulates an image, extending Binary
.
- max_width (
int
) – the maximum width of the image - max_height (
int
) – the maximum height of the image - verify_resolution (
bool
) – whether the image resolution should be verified to ensure it doesn’t go over the maximum image resolution (default:True
). Seeodoo.tools.image.ImageProcess
for maximum image resolution (default:45e6
).
class odoo.fields.Monetary[source]
Encapsulates a float
expressed in a given
res_currency
.
The decimal precision and currency symbol are taken from the currency_field
attribute.
class odoo.fields.Selection[source]
Encapsulates an exclusive choice between different values.
- selection (
list
(
tuple
(
str
,
str
)
) or
callable
or
str
) – specifies the possible values for this field. It is given as either a list of pairs(value, label)
, or a model method, or a method name. - selection_add (
list
(
tuple
(
str
,
str
)
)
) –provides an extension of the selection in the case of an overridden field. It is a list of pairs
(value, label)
or singletons(value,)
, where singleton values must appear in the overridden selection. The new values are inserted in an order that is consistent with the overridden selection and this list:selection = [('a', 'A'), ('b', 'B')] selection_add = [('c', 'C'), ('b',)] > result = [('a', 'A'), ('c', 'C'), ('b', 'B')]
The attribute selection
is mandatory except in the case of
related
or extended fields.
class odoo.fields.Text[source]
Very similar to Char
but used for longer contents, does not
have a size and usually displayed as a multiline text box.
bool
or
callable
) – enable the translation of the field’s values; use
translate=True
to translate field values as a whole; translate
may also be a callable such that translate(callback, value)
translates value
by using callback(term)
to retrieve the
translation of terms.Date(time) Fields
Dates
and Datetimes
are very important fields in any kind of business application.
Their misuse can create invisible yet painful bugs, this section
aims to provide Odoo developers with the knowledge required
to avoid misusing these fields.
When assigning a value to a Date/Datetime field, the following options are valid:
- A [UNKNOWN NODE title_reference] or [UNKNOWN NODE title_reference] object.
A string in the proper server format:
- [UNKNOWN NODE title_reference] or [UNKNOWN NODE title_reference].
The Date and Datetime fields class have helper methods to attempt conversion into a compatible type:
to_date()
will convert to adatetime.date
to_datetime()
will convert to adatetime.datetime
.
Example
To parse date/datetimes coming from external sources:
fields.Date.to_date(self._context.get('date_from'))
Date / Datetime comparison best practices:
- Date fields can only be compared to date objects.
- Datetime fields can only be compared to datetime objects.
Warning
Strings representing dates and datetimes can be compared between each other, however the result may not be the expected result, as a datetime string will always be greater than a date string, therefore this practice is heavily discouraged.
Common operations with dates and datetimes such as addition, substraction or
fetching the start/end of a period are exposed through both
Date
and Datetime
.
These helpers are also available by importing [UNKNOWN NODE title_reference].
Note
Timezones
Datetime fields are stored as [UNKNOWN NODE title_reference] columns in the database and are stored in the UTC timezone. This is by design, as it makes the Odoo database independent from the timezone of the hosting server system. Timezone conversion is managed entirely by the client side.
class odoo.fields.Date[source]
Encapsulates a python date
object.
static add(value, *args, **kwargs)[source]
Return the sum of value
and a relativedelta
.
- value – initial date or datetime.
- args – positional args to pass directly to
relativedelta
. - kwargs – keyword args to pass directly to
relativedelta
.
static context_today(record, timestamp=None)[source]
Return the current date as seen in the client’s timezone in a format fit for date fields.
Note
This method may be used to compute default values.
- record – recordset from which the timezone will be obtained.
- timestamp (
datetime
) – optional datetime value to use instead of the current date and time (must be a datetime, regular dates can’t be converted between timezones).
static end_of(value, granularity)[source]
Get end of a time period from a date or a datetime.
- value – initial date or datetime.
- granularity – Type of period in string, can be year, quarter, month, week, day or hour.
static start_of(value, granularity)[source]
Get start of a time period from a date or a datetime.
- value – initial date or datetime.
- granularity – type of period in string, can be year, quarter, month, week, day or hour.
static subtract(value, *args, **kwargs)[source]
Return the difference between value
and a relativedelta
.
- value – initial date or datetime.
- args – positional args to pass directly to
relativedelta
. - kwargs – keyword args to pass directly to
relativedelta
.
static to_date(value)[source]
Attempt to convert value
to a date
object.
Warning
If a datetime object is given as value, it will be converted to a date object and all datetime-specific information will be lost (HMS, TZ, …).
static to_string(value)[source]
Convert a date
or datetime
object to a string.
value
in the server’s date format, if value
is of
type datetime
, the hours, minute, seconds, tzinfo will be truncated.static today(*args)[source]
Return the current day in the format expected by the ORM.
Note
This function may be used to compute default values.
class odoo.fields.Datetime[source]
Encapsulates a python datetime
object.
static add(value, *args, **kwargs)[source]
Return the sum of value
and a relativedelta
.
- value – initial date or datetime.
- args – positional args to pass directly to
relativedelta
. - kwargs – keyword args to pass directly to
relativedelta
.
static context_timestamp(record, timestamp)[source]
Return the given timestamp converted to the client’s timezone.
Note
This method is not meant for use as a default initializer,
because datetime fields are automatically converted upon
display on client side. For default values, now()
should be used instead.
- record – recordset from which the timezone will be obtained.
- timestamp (
datetime
) – naive datetime value (expressed in UTC) to be converted to the client timezone.
static end_of(value, granularity)[source]
Get end of a time period from a date or a datetime.
- value – initial date or datetime.
- granularity – Type of period in string, can be year, quarter, month, week, day or hour.
static now(*args)[source]
Return the current day and time in the format expected by the ORM.
Note
This function may be used to compute default values.
static start_of(value, granularity)[source]
Get start of a time period from a date or a datetime.
- value – initial date or datetime.
- granularity – type of period in string, can be year, quarter, month, week, day or hour.
static subtract(value, *args, **kwargs)[source]
Return the difference between value
and a relativedelta
.
- value – initial date or datetime.
- args – positional args to pass directly to
relativedelta
. - kwargs – keyword args to pass directly to
relativedelta
.
static to_datetime(value)[source]
Convert an ORM value
into a datetime
value.
static to_string(value)[source]
Convert a datetime
or date
object to a string.
datetime
or
date
) – value to convert.value
in the server’s datetime format,
if value
is of type date
,
the time portion will be midnight (00:00:00).static today(*args)[source]
Return the current day, at midnight (00:00:00).
Relational Fields
class odoo.fields.Many2one[source]
The value of such a field is a recordset of size 0 (no record) or 1 (a single record).
- comodel_name (
str
) – name of the target modelMandatory
except for related or extended fields. - domain – an optional domain to set on candidate values on the client side (domain or string)
- context (
dict
) – an optional context to use on the client side when handling that field - ondelete (
str
) – what to do when the referred record is deleted; possible values are:'set null'
,'restrict'
,'cascade'
- auto_join (
bool
) – whether JOINs are generated upon search through that field (default:False
) - delegate (
bool
) – set it toTrue
to make fields of the target model accessible from the current model (corresponds to_inherits
) - check_company (
bool
) – Mark the field to be verified in_check_company()
. Add a default company domain depending on the field attributes.
class odoo.fields.One2many[source]
One2many field; the value of such a field is the recordset of all the
records in comodel_name
such that the field inverse_name
is equal to
the current record.
- comodel_name (
str
) – name of the target model - inverse_name (
str
) – name of the inverseMany2one
field incomodel_name
- domain – an optional domain to set on candidate values on the client side (domain or string)
- context (
dict
) – an optional context to use on the client side when handling that field - auto_join (
bool
) – whether JOINs are generated upon search through that field (default:False
) - limit (
int
) – optional limit to use upon read
The attributes comodel_name
and inverse_name
are mandatory except in
the case of related fields or field extensions.
class odoo.fields.Many2many[source]
Many2many field; the value of such a field is the recordset.
- comodel_name – name of the target model (string) mandatory except in the case of related or extended fields
- relation (
str
) – optional name of the table that stores the relation in the database - column1 (
str
) – optional name of the column referring to “these” records in the tablerelation
- column2 (
str
) – optional name of the column referring to “those” records in the tablerelation
The attributes relation
, column1
and column2
are optional.
If not given, names are automatically generated from model names,
provided model_name
and comodel_name
are different!
Note that having several fields with implicit relation parameters on a given model with the same comodel is not accepted by the ORM, since those field would use the same table. The ORM prevents two many2many fields to use the same relation parameters, except if
- both fields use the same model, comodel, and relation parameters are explicit; or
- at least one field belongs to a model with
_auto = False
.
- domain – an optional domain to set on candidate values on the client side (domain or string)
- context (
dict
) – an optional context to use on the client side when handling that field - check_company (
bool
) – Mark the field to be verified in_check_company()
. Add a default company domain depending on the field attributes. - limit (
int
) – optional limit to use upon read
Pseudo-relational fields
class odoo.fields.Reference[source]
Pseudo-relational field (no FK in database).
The field value is stored as a string
following the pattern
"res_model.res_id"
in database.
class odoo.fields.Many2oneReference[source]
Pseudo-relational field (no FK in database).
The field value is stored as an integer
id in database.
Contrary to Reference
fields, the model has to be specified
in a Char
field, whose name has to be specified in the
[UNKNOWN NODE title_reference] attribute for the current Many2oneReference
field.
Computed Fields
Fields can be computed (instead of read straight from the database) using the
compute
parameter. It must assign the computed value to the field. If
it uses the values of other fields, it should specify those fields using
depends()
.
from odoo import api
total = fields.Float(compute='_compute_total')
@api.depends('value', 'tax')
def _compute_total(self):
for record in self:
record.total = record.value + record.value * record.tax
dependencies can be dotted paths when using sub-fields:
@api.depends('line_ids.value') def _compute_total(self): for record in self: record.total = sum(line.value for line in record.line_ids)
- computed fields are not stored by default, they are computed and
returned when requested. Setting
store=True
will store them in the database and automatically enable searching. searching on a computed field can also be enabled by setting the
search
parameter. The value is a method name returning a Search domains.upper_name = field.Char(compute='_compute_upper', search='_search_upper') def _search_upper(self, operator, value): if operator == 'like': operator = 'ilike' return [('name', operator, value)]
The search method is invoked when processing domains before doing an actual search on the model. It must return a domain equivalent to the condition:
field operator value
.
Computed fields are readonly by default. To allow setting values on a computed field, use the
inverse
parameter. It is the name of a function reversing the computation and setting the relevant fields:document = fields.Char(compute='_get_document', inverse='_set_document') def _get_document(self): for record in self: with open(record.get_document_path) as f: record.document = f.read() def _set_document(self): for record in self: if not record.document: continue with open(record.get_document_path()) as f: f.write(record.document)
multiple fields can be computed at the same time by the same method, just use the same method on all fields and set all of them:
discount_value = fields.Float(compute='_apply_discount') total = fields.Float(compute='_apply_discount') @api.depends('value', 'discount') def _apply_discount(self): for record in self: # compute actual discount from discount percentage discount = record.value * record.discount record.discount_value = discount record.total = record.value - discount
Automatic fields
odoo.fields.id
Identifier field
If length of current recordset is 1, return id of unique record in it.
Raise an Error otherwise.
odoo.fields.create_date
odoo.fields.create_uid
odoo.fields.write_date
odoo.fields.write_uid
Reserved Field names
A few field names are reserved for pre-defined behaviors beyond that of automated fields. They should be defined on a model when the related behavior is desired:
odoo.fields.name
default value for _rec_name
, used to
display records in context where a representative “naming” is
necessary.
odoo.fields.active
toggles the global visibility of the record, if active
is set to
False
the record is invisible in most searches and listing.
odoo.fields.state
lifecycle stages of the object, used by the states
attribute on
fields
.
odoo.fields.parent_id
default_value of _parent_name
, used to organize
records in a tree structure and enables the child_of
and parent_of
operators in domains.
odoo.fields.parent_path
When _parent_store
is set to True, used to store a value reflecting
the tree structure of _parent_name
, and to optimize the operators
child_of
and parent_of
in search domains.
It must be declared with index=True
for proper operation.
odoo.fields.company_id
Main field name used for Odoo multi-company behavior.
Used by [UNKNOWN NODE title_reference] to check multi company consistency. Defines whether a record is shared between companies (no value) or only accessible by the users of a given company.
Many2one
:type: res_company
Recordsets
Interactions with models and records are performed through recordsets, an ordered collection of records of the same model.
Warning
Contrary to what the name implies, it is currently possible for recordsets to contain duplicates. This may change in the future.
Methods defined on a model are executed on a recordset, and their self
is
a recordset:
class AModel(models.Model):
_name = 'a.model'
def a_method(self):
# self can be anything between 0 records and all records in the
# database
self.do_operation()
Iterating on a recordset will yield new sets of a single record (“singletons”), much like iterating on a Python string yields strings of a single characters:
def do_operation(self):
print(self) # => a.model(1, 2, 3, 4, 5)
for record in self:
print(record) # => a.model(1), then a.model(2), then a.model(3), ...
Field access
Recordsets provide an “Active Record” interface: model fields can be read and written directly from the record as attributes.
Note
When accessing non-relational fields on a recordset of potentially multiple
records, use mapped()
:
total_qty = sum(self.mapped('qty'))
Field values can also be accessed like dict items, which is more elegant and
safer than getattr()
for dynamic field names.
Setting a field’s value triggers an update to the database:
>>> record.name
Example Name
>>> record.company_id.name
Company Name
>>> record.name = "Bob"
>>> field = "name"
>>> record[field]
Bob
Warning
Trying to read a field on multiple records will raise an error for non relational fields.
Accessing a relational field (Many2one
,
One2many
, Many2many
)
always returns a recordset, empty if the field is not set.
Record cache and prefetching
Odoo maintains a cache for the fields of the records, so that not every field access issues a database request, which would be terrible for performance. The following example queries the database only for the first statement:
record.name # first access reads value from database
record.name # second access gets value from cache
To avoid reading one field on one record at a time, Odoo prefetches records and fields following some heuristics to get good performance. Once a field must be read on a given record, the ORM actually reads that field on a larger recordset, and stores the returned values in cache for later use. The prefetched recordset is usually the recordset from which the record comes by iteration. Moreover, all simple stored fields (boolean, integer, float, char, text, date, datetime, selection, many2one) are fetched altogether; they correspond to the columns of the model’s table, and are fetched efficiently in the same query.
Consider the following example, where partners
is a recordset of 1000
records. Without prefetching, the loop would make 2000 queries to the database.
With prefetching, only one query is made:
for partner in partners:
print partner.name # first pass prefetches 'name' and 'lang'
# (and other fields) on all 'partners'
print partner.lang
The prefetching also works on secondary records: when relational fields are read, their values (which are records) are subscribed for future prefetching. Accessing one of those secondary records prefetches all secondary records from the same model. This makes the following example generate only two queries, one for partners and one for countries:
countries = set()
for partner in partners:
country = partner.country_id # first pass prefetches all partners
countries.add(country.name) # first pass prefetches all countries
Method decorators
The Odoo API module defines Odoo Environments and method decorators.
odoo.api.depends(*args)[source]
Return a decorator that specifies the field dependencies of a “compute” method (for new-style function fields). Each argument must be a string that consists in a dot-separated sequence of field names:
pname = fields.Char(compute='_compute_pname')
@api.depends('partner_id.name', 'partner_id.is_company')
def _compute_pname(self):
for record in self:
if record.partner_id.is_company:
record.pname = (record.partner_id.name or "").upper()
else:
record.pname = record.partner_id.name
One may also pass a single function as argument. In that case, the dependencies are given by calling the function with the field’s model.
odoo.api.depends_context(*args)[source]
Return a decorator that specifies the context dependencies of a non-stored “compute” method. Each argument is a key in the context’s dictionary:
price = fields.Float(compute='_compute_product_price')
@api.depends_context('pricelist')
def _compute_product_price(self):
for product in self:
if product.env.context.get('pricelist'):
pricelist = self.env['product.pricelist'].browse(product.env.context['pricelist'])
else:
pricelist = self.env['product.pricelist'].get_default_pricelist()
product.price = pricelist.get_products_price(product).get(product.id, 0.0)
All dependencies must be hashable. The following keys have special support:
- [UNKNOWN NODE title_reference] (value in context or current company id),
- [UNKNOWN NODE title_reference] (current user id and superuser flag),
- [UNKNOWN NODE title_reference] (value in env.context or value in field.context).
odoo.api.constrains(*args)[source]
Decorate a constraint checker.
Each argument must be a field name used in the check:
@api.constrains('name', 'description')
def _check_description(self):
for record in self:
if record.name == record.description:
raise ValidationError("Fields name and description must be different")
Invoked on the records on which one of the named fields has been modified.
Should raise ValidationError
if the
validation failed.
Warning
@constrains
only supports simple field names, dotted names
(fields of relational fields e.g. partner_id.customer
) are not
supported and will be ignored.
@constrains
will be triggered only if the declared fields in the
decorated method are included in the create
or write
call.
It implies that fields not present in a view will not trigger a call
during a record creation. A override of create
is necessary to make
sure a constraint will always be triggered (e.g. to test the absence of
value).
odoo.api.onchange(*args)[source]
Return a decorator to decorate an onchange method for given fields.
In the form views where the field appears, the method will be called when one of the given fields is modified. The method is invoked on a pseudo-record that contains the values present in the form. Field assignments on that record are automatically sent back to the client.
Each argument must be a field name:
@api.onchange('partner_id')
def _onchange_partner(self):
self.message = "Dear %s" % (self.partner_id.name or "")
return {
'domain': {'other_id': [('partner_id', '=', partner_id)]},
'warning': {'title': "Warning", 'message': "What is this?", 'type': 'notification'},
}
If the type is set to notification, the warning will be displayed in a notification. Otherwise it will be displayed in a dialog as default.
Warning
@onchange
only supports simple field names, dotted names
(fields of relational fields e.g. partner_id.tz
) are not
supported and will be ignored
Danger
Since @onchange
returns a recordset of pseudo-records,
calling any one of the CRUD methods
(create()
, read()
, write()
, unlink()
)
on the aforementioned recordset is undefined behaviour,
as they potentially do not exist in the database yet.
Instead, simply set the record’s field like shown in the example
above or call the update()
method.
Warning
It is not possible for a one2many
or many2many
field to modify
itself via onchange. This is a webclient limitation - see #2693.
odoo.api.returns(model, downgrade=None, upgrade=None)[source]
Return a decorator for methods that return instances of model
.
- model – a model name, or
'self'
for the current model - downgrade – a function
downgrade(self, value, *args, **kwargs)
to convert the record-stylevalue
to a traditional-style output - upgrade – a function
upgrade(self, value, *args, **kwargs)
to convert the traditional-stylevalue
to a record-style output
The arguments self
, *args
and **kwargs
are the ones passed
to the method in the record-style.
The decorator adapts the method output to the api style: id
, ids
or
False
for the traditional style, and recordset for the record style:
@model
@returns('res.partner')
def find_partner(self, arg):
... # return some record
# output depends on call style: traditional vs record style
partner_id = model.find_partner(cr, uid, arg, context=context)
# recs = model.browse(cr, uid, ids, context)
partner_record = recs.find_partner(arg)
Note that the decorated method must satisfy that convention.
Those decorators are automatically inherited: a method that overrides
a decorated existing method will be decorated with the same
@returns(model)
.
odoo.api.model(method)[source]
Decorate a record-style method where self
is a recordset, but its
contents is not relevant, only the model is. Such a method:
@api.model
def method(self, args):
...
odoo.api.model_create_multi(method)[source]
Decorate a method that takes a list of dictionaries and creates multiple records. The method may be called with either a single dict or a list of dicts:
record = model.create(vals)
records = model.create([vals, ...])
Environment
The Environment
stores various contextual data used by
the ORM: the database cursor (for database queries), the current user
(for access rights checking) and the current context (storing arbitrary
metadata). The environment also stores caches.
All recordsets have an environment, which is immutable, can be accessed
using env
and gives access to:
- the current user (
user
) - the cursor (
cr
) - the superuser flag (
su
) - or the context (
context
)
>>> records.env
<Environment object ...>
>>> records.env.user
res.user(3)
>>> records.env.cr
<Cursor object ...)
When creating a recordset from an other recordset, the environment is inherited. The environment can be used to get an empty recordset in an other model, and query that model:
>>> self.env['res.partner']
res.partner()
>>> self.env['res.partner'].search([['is_company', '=', True], ['customer', '=', True]])
res.partner(7, 18, 12, 14, 17, 19, 8, 31, 26, 16, 13, 20, 30, 22, 29, 15, 23, 28, 74)
Environment.ref(xml_id, raise_if_not_found=True)[source]
Return the record corresponding to the given xml_id
.
Environment.lang
Return the current language code.
Environment.user
Return the current user (as an instance).
res_users
Environment.company
Return the current company (as an instance).
If not specified in the context ([UNKNOWN NODE title_reference]), fallback on current user main company.
Warning
No sanity checks applied in sudo mode ! When in sudo mode, a user can access any company, even if not in his allowed companies.
This allows to trigger inter-company modifications, even if the current user doesn’t have access to the targeted company.
Environment.companies
Return a recordset of the enabled companies by the user.
If not specified in the context([UNKNOWN NODE title_reference]), fallback on current user companies.
Warning
No sanity checks applied in sudo mode ! When in sudo mode, a user can access any company, even if not in his allowed companies.
This allows to trigger inter-company modifications, even if the current user doesn’t have access to the targeted company.
Altering the environment
Model.with_context([context][, **overrides]) → records[source]
Returns a new version of this recordset attached to an extended context.
The extended context is either the provided context
in which
overrides
are merged or the current context in which
overrides
are merged e.g.:
# current context is {'key1': True}
r2 = records.with_context({}, key2=True)
# -> r2._context is {'key2': True}
r2 = records.with_context(key2=True)
# -> r2._context is {'key1': True, 'key2': True}
Model.with_user(user)[source]
Return a new version of this recordset attached to the given user, in non-superuser mode, unless [UNKNOWN NODE title_reference] is the superuser (by convention, the superuser is always in superuser mode.)
Model.with_env(env)[source]
Return a new version of this recordset attached to the provided environment.
Environment
) – Warning
The new environment will not benefit from the current
environment’s data cache, so later data access may incur extra
delays while re-fetching from the database.
The returned recordset has the same prefetch object as self
.
Model.sudo([flag=True])[source]
Returns a new version of this recordset with superuser mode enabled or disabled, depending on [UNKNOWN NODE title_reference]. The superuser mode does not change the current user, and simply bypasses access rights checks.
Warning
Using sudo
could cause data access to cross the
boundaries of record rules, possibly mixing records that
are meant to be isolated (e.g. records from different
companies in multi-company environments).
It may lead to un-intuitive results in methods which select one record among many - for example getting the default company, or selecting a Bill of Materials.
Note
Because the record rules and access control will have to be
re-evaluated, the new recordset will not benefit from the current
environment’s data cache, so later data access may incur extra
delays while re-fetching from the database.
The returned recordset has the same prefetch object as self
.
SQL Execution
The cr
attribute on environments is the
cursor for the current database transaction and allows executing SQL directly,
either for queries which are difficult to express using the ORM (e.g. complex
joins) or for performance reasons:
self.env.cr.execute("some_sql", param1, param2, param3)
Because models use the same cursor and the Environment
holds various caches, these caches must be invalidated when altering the
database in raw SQL, or further uses of models may become incoherent. It is
necessary to clear caches when using CREATE
, UPDATE
or DELETE
in
SQL, but not SELECT
(which simply reads the database).
Note
Clearing caches can be performed using the
invalidate_cache()
method.
Model.invalidate_cache(fnames=None, ids=None)[source]
Invalidate the record caches after some records have been modified.
If both fnames
and ids
are None
, the whole cache is cleared.
- fnames – the list of modified fields, or
None
for all fields - ids – the list of modified record ids, or
None
for all
Warning
Executing raw SQL bypasses the ORM, and by consequent, Odoo security rules. Please make sure your queries are sanitized when using user input and prefer using ORM utilities if you don’t really need to use SQL queries.
Common ORM methods
Create/update
Model.create(vals_list) → records[source]
Creates new records for the model.
The new records are initialized using the values from the list of dicts
vals_list
, and if necessary those from default_get()
.
list
) – values for the model’s fields, as a list of dictionaries:
[{'field_name': field_value, ...}, ...]
For backward compatibility, vals_list
may be a dictionary.
It is treated as a singleton list [vals]
, and a single record
is returned.
see write()
for details
- AccessError –
- if user has no create rights on the requested object
- if user tries to bypass access rules for create on the requested object
- ValidationError – if user tries to enter invalid value for a field that is not in selection
- UserError – if a loop would be created in a hierarchy of objects a result of the operation (such as setting an object as its own parent)
Model.copy(default=None)[source]
Duplicate record self
updating it with default values
dict
) – dictionary of field values to override in the
original values of the copied record, e.g: {'field_name': overridden_value, ...}
Model.default_get(fields) → default_values[source]
Return default values for the fields in fields_list
. Default
values are determined by the context, user defaults, and the model
itself.
Model.name_create(name) → record[source]
Create a new record by calling create()
with only one value
provided: the display name of the new record.
The new record will be initialized with any default values
applicable to this model, or provided through the context. The usual
behavior of create()
applies.
name_get()
pair value of the created recordModel.write(vals)[source]
Updates all records in the current set with the provided values.
dict
) – fields to update and the value to set on them e.g:
{'foo': 1, 'bar': "Qux"}
will set the field foo
to 1
and the field bar
to
"Qux"
if those are valid (otherwise it will trigger an error).
- AccessError –
- if user has no write rights on the requested object
- if user tries to bypass access rules for write on the requested object
- ValidationError – if user tries to enter invalid value for a field that is not in selection
- UserError – if a loop would be created in a hierarchy of objects a result of the operation (such as setting an object as its own parent)
- For numeric fields (
Integer
,Float
) the value should be of the corresponding type - For
Boolean
, the value should be abool
- For
Selection
, the value should match the selection values (generallystr
, sometimesint
) - For
Many2one
, the value should be the database identifier of the record to set Other non-relational fields use a string for value
One2many
andMany2many
use a special “commands” format to manipulate the set of records stored in/associated with the field.This format is a list of triplets executed sequentially, where each triplet is a command to execute on the set of records. Not all commands apply in all situations. Possible commands are:
(0, 0, values)
- adds a new record created from the provided
value
dict. (1, id, values)
- updates an existing record of id
id
with the values invalues
. Can not be used increate()
. (2, id, 0)
- removes the record of id
id
from the set, then deletes it (from the database). Can not be used increate()
. (3, id, 0)
- removes the record of id
id
from the set, but does not delete it. Can not be used increate()
. (4, id, 0)
- adds an existing record of id
id
to the set. (5, 0, 0)
- removes all records from the set, equivalent to using the
command
3
on every record explicitly. Can not be used increate()
. (6, 0, ids)
- replaces all existing records in the set by the
ids
list, equivalent to using the command5
followed by a command4
for eachid
inids
.
Model.flush(fnames=None, records=None)[source]
Process all the pending recomputations (or at least the given field names [UNKNOWN NODE title_reference] if present) and flush the pending updates to the database.
Search/Read
Model.browse([ids]) → records[source]
Returns a recordset for the ids provided as parameter in the current environment.
self.browse([7, 18, 12])
res.partner(7, 18, 12)
Model.search(args[, offset=0][, limit=None][, order=None][, count=False])[source]
Searches for records based on the args
search domain.
- args – A search domain. Use an empty list to match all records.
- offset (
int
) – number of results to ignore (default: none) - limit (
int
) – maximum number of records to return (default: all) - order (
str
) – sort string - count (
bool
) – if True, only counts and returns the number of matching records (default: False)
limit
records matching the search criteria- if user tries to bypass access rules for read on the requested object.
Model.search_count(args) → int[source]
Returns the number of records in the current model matching the provided domain.
Model.name_search(name='', args=None, operator='ilike', limit=100) → records[source]
Search for records that have a display name matching the given
name
pattern when compared with the given operator
, while also
matching the optional search domain (args
).
This is used for example to provide suggestions based on a partial
value for a relational field. Sometimes be seen as the inverse
function of name_get()
, but it is not guaranteed to be.
This method is equivalent to calling search()
with a search
domain based on display_name
and then name_get()
on the
result of the search.
(id, text_repr)
for all matching records.Model.read([fields])[source]
Reads the requested fields for the records in self
, low-level/RPC
method. In Python code, prefer browse()
.
Model.read_group(domain, fields, groupby, offset=0, limit=None, orderby=False, lazy=True)[source]
Get the list of records in list view grouped by the given groupby
fields.
- domain (
list
) – A search domain. Use an empty list to match all records. - fields (
list
) – list of fields present in the list view specified on the object. Each element is either ‘field’ (field name, using the default aggregation), or ‘field:agg’ (aggregate field with aggregation function ‘agg’), or ‘name:agg(field)’ (aggregate field with ‘agg’ and return it as ‘name’). The possible aggregation functions are the ones provided by PostgreSQL (https://www.postgresql.org/docs/current/static/functions-aggregate.html) and ‘count_distinct’, with the expected meaning. - groupby (
list
) – list of groupby descriptions by which the records will be grouped. A groupby description is either a field (then it will be grouped by that field) or a string ‘field:groupby_function’. Right now, the only functions supported are ‘day’, ‘week’, ‘month’, ‘quarter’ or ‘year’, and they only make sense for date/datetime fields. - offset (
int
) – optional number of records to skip - limit (
int
) – optional max number of records to return - orderby (
list
) – optionalorder by
specification, for overriding the natural sort ordering of the groups, see alsosearch()
(supported only for many2one fields currently) - lazy (
bool
) – if true, the results are only grouped by the first groupby and the remaining groupbys are put in the __context key. If false, all the groupbys are done in one call.
list of dictionaries(one dictionary for each record) containing:
- the values of fields grouped by the fields in
groupby
argument - __domain: list of tuples specifying the search criteria
- __context: dictionary with argument like
groupby
- if user has no read rights on the requested object
- if user tries to bypass access rules for read on the requested object
Fields/Views
Model.fields_get([fields][, attributes])[source]
Return the definition of each field.
The returned value is a dictionary (indexed by field name) of dictionaries. The _inherits’d fields are included. The string, help, and selection (if present) attributes are translated.
- allfields – list of fields to document, all if empty or not provided
- attributes – list of description attributes to return for each field, all if empty or not provided
Model.fields_view_get([view_id | view_type='form'])[source]
Get the detailed composition of the requested view like fields, model, view architecture
- AttributeError –
- if the inherited view has unknown position to work with other than ‘before’, ‘after’, ‘inside’, ‘replace’
- if some tag other than ‘position’ is found in parent view
- Invalid ArchitectureError – if there is view type other than form, tree, calendar, search etc defined on the structure
Search domains
A domain is a list of criteria, each criterion being a triple (either a
list
or a tuple
) of (field_name, operator, value)
where:
field_name
(str
)- a field name of the current model, or a relationship traversal through
a
Many2one
using dot-notation e.g.'street'
or'partner_id.country'
operator
(str
)an operator used to compare the
field_name
with thevalue
. Valid operators are:=
- equals to
!=
- not equals to
>
- greater than
>=
- greater than or equal to
<
- less than
<=
- less than or equal to
=?
- unset or equals to (returns true if
value
is eitherNone
orFalse
, otherwise behaves like=
) =like
- matches
field_name
against thevalue
pattern. An underscore_
in the pattern stands for (matches) any single character; a percent sign%
matches any string of zero or more characters. like
- matches
field_name
against the%value%
pattern. Similar to=like
but wrapsvalue
with ‘%’ before matching not like
- doesn’t match against the
%value%
pattern ilike
- case insensitive
like
not ilike
- case insensitive
not like
=ilike
- case insensitive
=like
in
- is equal to any of the items from
value
,value
should be a list of items not in
- is unequal to all of the items from
value
child_of
is a child (descendant) of a
value
record (value can be either one item or a list of items).Takes the semantics of the model into account (i.e following the relationship field named by
_parent_name
).parent_of
is a parent (ascendant) of a
value
record (value can be either one item or a list of items).Takes the semantics of the model into account (i.e following the relationship field named by
_parent_name
).
value
- variable type, must be comparable (through
operator
) to the named field.
Domain criteria can be combined using logical operators in prefix form:
'&'
- logical AND, default operation to combine criteria following one another. Arity 2 (uses the next 2 criteria or combinations).
'|'
- logical OR, arity 2.
'!'
logical NOT, arity 1.
Note
Mostly to negate combinations of criteria Individual criterion generally have a negative form (e.g.
=
->!=
,<
->>=
) which is simpler than negating the positive.
Example
To search for partners named ABC, from belgium or germany, whose language is not english:
[('name','=','ABC'),
('language.code','!=','en_US'),
'|',('country_id.code','=','be'),
('country_id.code','=','de')]
This domain is interpreted as:
(name is 'ABC')
AND (language is NOT english)
AND (country is Belgium OR Germany)
Unlink
Model.unlink()[source]
Deletes the records of the current set
- AccessError –
- if user has no unlink rights on the requested object
- if user tries to bypass access rules for unlink on the requested object
- UserError – if the record is default property for other records
Record(set) information
Model.ids
Return the list of actual record ids corresponding to self
.
odoo.models.env
Returns the environment of the given recordset.
Environment
Model.exists() → records[source]
Returns the subset of records in self
that exist, and marks deleted
records as such in cache. It can be used as a test on records:
if record.exists():
...
By convention, new records are returned as existing.
Model.ensure_one()[source]
Verify that the current recorset holds a single record.
len(self) != 1
Model.name_get() → [(id, name), ...][source]
Returns a textual representation for the records in self
.
By default this is the value of the display_name
field.
(id, text_repr)
for each recordsModel.get_metadata()[source]
Return some metadata about the given records.
list of dictionaries with the following keys:
- id: object id
- create_uid: user who created the record
- create_date: date when the record was created
- write_uid: last user who changed the record
- write_date: date of the last change to the record
- xmlid: XML ID to use to refer to this record (if there is one), in format
module.name
- noupdate: A boolean telling if the record will be updated or not
Operations
Recordsets are immutable, but sets of the same model can be combined using various set operations, returning new recordsets.
record in set
returns whetherrecord
(which must be a 1-element recordset) is present inset
.record not in set
is the inverse operationset1 <= set2
andset1 < set2
return whetherset1
is a subset ofset2
(resp. strict)set1 >= set2
andset1 > set2
return whetherset1
is a superset ofset2
(resp. strict)set1 | set2
returns the union of the two recordsets, a new recordset containing all records present in either sourceset1 & set2
returns the intersection of two recordsets, a new recordset containing only records present in both sourcesset1 - set2
returns a new recordset containing only records ofset1
which are not inset2
Recordsets are iterable so the usual Python tools are available for
transformation (map()
, sorted()
,
ifilter()
, …) however these return either a
list
or an iterator, removing the ability to
call methods on their result, or to use set operations.
Recordsets therefore provide the following operations returning recordsets themselves (when possible):
Filter
Model.filtered(func)[source]
Return the records in self
satisfying func
.
# only keep records whose company is the current user's
records.filtered(lambda r: r.company_id == user.company_id)
# only keep records whose partner is a company
records.filtered("partner_id.is_company")
Model.filtered_domain(domain)[source]
Map
Model.mapped(func)[source]
Apply func
on all records in self
, and return the result as a
list or a recordset (if func
return recordsets). In the latter
case, the order of the returned recordset is arbitrary.
self
records.# returns a list of summing two fields for each record in the set
records.mapped(lambda r: r.field1 + r.field2)
The provided function can be a string to get field values:
# returns a list of names
records.mapped('name')
# returns a recordset of partners
records.mapped('partner_id')
# returns the union of all partner banks, with duplicates removed
records.mapped('partner_id.bank_ids')
Note
Since V13, multi-relational field access is supported and works like a mapped call:
records.partner_id # == records.mapped('partner_id')
records.partner_id.bank_ids # == records.mapped('partner_id.bank_ids')
records.partner_id.mapped('name') # == records.mapped('partner_id.name')
Sort
Model.sorted(key=None, reverse=False)[source]
Return the recordset self
ordered by key
.
# sort records by name
records.sorted(key=lambda r: r.name)
Inheritance and extension
Odoo provides three different mechanisms to extend models in a modular way:
- creating a new model from an existing one, adding new information to the copy but leaving the original module as-is
- extending models defined in other modules in-place, replacing the previous version
- delegating some of the model’s fields to records it contains
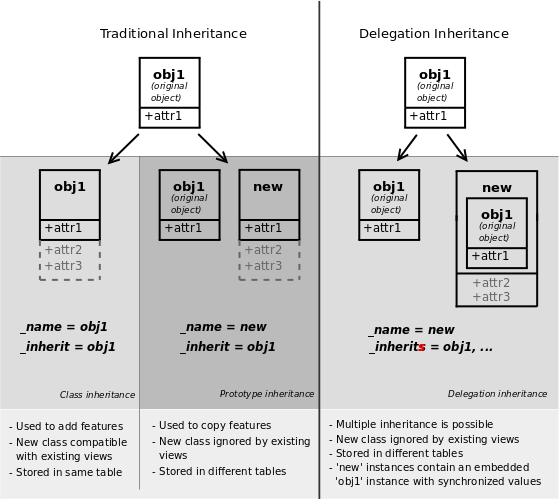
Classical inheritance
When using the _inherit
and
_name
attributes together, Odoo creates a new
model using the existing one (provided via
_inherit
) as a base. The new model gets all the
fields, methods and meta-information (defaults & al) from its base.
class Inheritance0(models.Model):
_name = 'inheritance.0'
_description = 'Inheritance Zero'
name = fields.Char()
def call(self):
return self.check("model 0")
def check(self, s):
return "This is {} record {}".format(s, self.name)
class Inheritance1(models.Model):
_name = 'inheritance.1'
_inherit = 'inheritance.0'
_description = 'Inheritance One'
def call(self):
return self.check("model 1")
and using them:
a = env['inheritance.0'].create({'name': 'A'})
b = env['inheritance.1'].create({'name': 'B'})
a.call()
b.call()
will yield:
"This is model 0 record A"
"This is model 1 record B"
the second model has inherited from the first model’s check
method and its
name
field, but overridden the call
method, as when using standard
Python inheritance.
Extension
When using _inherit
but leaving out
_name
, the new model replaces the existing one,
essentially extending it in-place. This is useful to add new fields or methods
to existing models (created in other modules), or to customize or reconfigure
them (e.g. to change their default sort order):
class Extension0(models.Model):
_name = 'extension.0'
_description = 'Extension zero'
name = fields.Char(default="A")
class Extension1(models.Model):
_inherit = 'extension.0'
description = fields.Char(default="Extended")
record = env['extension.0'].create({})
record.read()[0]
will yield:
{'name': "A", 'description': "Extended"}
Note
it will also yield the various automatic fields unless they’ve been disabled
Delegation
The third inheritance mechanism provides more flexibility (it can be altered
at runtime) but less power: using the _inherits
a model delegates the lookup of any field not found on the current model
to “children” models. The delegation is performed via
Reference
fields automatically set up on the parent
model.
The main difference is in the meaning. When using Delegation, the model has one instead of is one, turning the relationship in a composition instead of inheritance:
class Screen(models.Model):
_name = 'delegation.screen'
_description = 'Screen'
size = fields.Float(string='Screen Size in inches')
class Keyboard(models.Model):
_name = 'delegation.keyboard'
_description = 'Keyboard'
layout = fields.Char(string='Layout')
class Laptop(models.Model):
_name = 'delegation.laptop'
_description = 'Laptop'
_inherits = {
'delegation.screen': 'screen_id',
'delegation.keyboard': 'keyboard_id',
}
name = fields.Char(string='Name')
maker = fields.Char(string='Maker')
# a Laptop has a screen
screen_id = fields.Many2one('delegation.screen', required=True, ondelete="cascade")
# a Laptop has a keyboard
keyboard_id = fields.Many2one('delegation.keyboard', required=True, ondelete="cascade")
record = env['delegation.laptop'].create({
'screen_id': env['delegation.screen'].create({'size': 13.0}).id,
'keyboard_id': env['delegation.keyboard'].create({'layout': 'QWERTY'}).id,
})
record.size
record.layout
will result in:
13.0
'QWERTY'
and it’s possible to write directly on the delegated field:
record.write({'size': 14.0})
Warning
when using delegation inheritance, methods are not inherited, only fields
Fields Incremental Definition
A field is defined as class attribute on a model class. If the model is extended, one can also extend the field definition by redefining a field with the same name and same type on the subclass. In that case, the attributes of the field are taken from the parent class and overridden by the ones given in subclasses.
For instance, the second class below only adds a tooltip on the field
state
:
class First(models.Model):
_name = 'foo'
state = fields.Selection([...], required=True)
class Second(models.Model):
_inherit = 'foo'
state = fields.Selection(help="Blah blah blah")
Error management
The Odoo Exceptions module defines a few core exception types.
Those types are understood by the RPC layer. Any other exception type bubbling until the RPC layer will be treated as a ‘Server error’.
Note
If you consider introducing new exceptions,
check out the odoo.addons.test_exceptions
module.
exception odoo.exceptions.AccessDenied(message='Access denied')[source]
Login/password error.
Note
No traceback.
Example
When you try to log with a wrong password.
exception odoo.exceptions.AccessError(msg)[source]
Access rights error.
Example
When you try to read a record that you are not allowed to.
exception odoo.exceptions.CacheMiss(record, field)[source]
Missing value(s) in cache.
Example
When you try to read a value in a flushed cache.
exception odoo.exceptions.MissingError(msg)[source]
Missing record(s).
Example
When you try to write on a deleted record.
exception odoo.exceptions.RedirectWarning[source]
Warning with a possibility to redirect the user instead of simply displaying the warning message.
exception odoo.exceptions.UserError(msg)[source]
Generic error managed by the client.
Typically when the user tries to do something that has no sense given the current state of a record.
exception odoo.exceptions.ValidationError(msg)[source]
Violation of python constraints.
Example
When you try to create a new user with a login which already exist in the db.